Validation Form in wiget
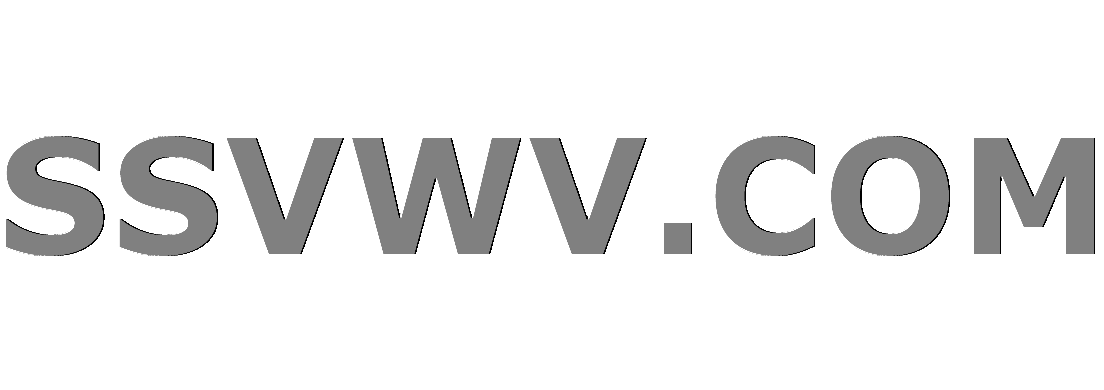
Multi tool use
up vote
1
down vote
favorite
I would like to have a form available on every page in my web app. So I created a widget with a form.
class AddNewURL extends Widget
public $model;
public function init()
parent::init();
if ($this->model === null)
$this->model = new ProductUrlForm();
public function run()
return $this->render('addNewURLForm', [
'model' => $this->model,
]);
and widget view
<div class="modal fade bs-example-modal-lg" id="newURL" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<?php Pjax::begin(); ?>
<?php $form = ActiveForm::begin([
'action' => Url::to(['site/new-url']),
'options' => ['data' => ['pjax' => true]],
]) ?>
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span>
</button>
<h4 class="modal-title" id="myModalLabel">Add new URL</h4>
</div>
<div class="modal-body">
<div class="row">
<div class="col-md-12">
<?= $form->field($model, 'url', ['errorOptions' => ['class' => 'help-block', 'encode' => false]])
->textInput(['maxlength' => false])
?>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Add</button>
</div>
<?php ActiveForm::end() ?>
<?php Pjax::end(); ?>
</div>
</div>
</div>
and this how I use this widget in my layouts/main.php
<?= appwidgetsAddNewURL::widget() ?>
in my site/new-url action I have
public function actionNewUrl()
$model = new ProductUrlForm();
$model->status = STATUS_NEW;
if ($model->load(Yii::$app->request->post()) && $model->save())
//return success message
return $this->render('index', [
'model' => $model,
]);
and now when I submit form data goes to my action, the form is reset and that's all. How to show validation error or success message using pjax? In my model I have few custom validation rules.
Maybe there is better solution to use one form on all pages?
forms yii2 widget pjax
add a comment |
up vote
1
down vote
favorite
I would like to have a form available on every page in my web app. So I created a widget with a form.
class AddNewURL extends Widget
public $model;
public function init()
parent::init();
if ($this->model === null)
$this->model = new ProductUrlForm();
public function run()
return $this->render('addNewURLForm', [
'model' => $this->model,
]);
and widget view
<div class="modal fade bs-example-modal-lg" id="newURL" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<?php Pjax::begin(); ?>
<?php $form = ActiveForm::begin([
'action' => Url::to(['site/new-url']),
'options' => ['data' => ['pjax' => true]],
]) ?>
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span>
</button>
<h4 class="modal-title" id="myModalLabel">Add new URL</h4>
</div>
<div class="modal-body">
<div class="row">
<div class="col-md-12">
<?= $form->field($model, 'url', ['errorOptions' => ['class' => 'help-block', 'encode' => false]])
->textInput(['maxlength' => false])
?>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Add</button>
</div>
<?php ActiveForm::end() ?>
<?php Pjax::end(); ?>
</div>
</div>
</div>
and this how I use this widget in my layouts/main.php
<?= appwidgetsAddNewURL::widget() ?>
in my site/new-url action I have
public function actionNewUrl()
$model = new ProductUrlForm();
$model->status = STATUS_NEW;
if ($model->load(Yii::$app->request->post()) && $model->save())
//return success message
return $this->render('index', [
'model' => $model,
]);
and now when I submit form data goes to my action, the form is reset and that's all. How to show validation error or success message using pjax? In my model I have few custom validation rules.
Maybe there is better solution to use one form on all pages?
forms yii2 widget pjax
how and where are you calling this widget? add to your question.
– Muhammad Omer Aslam
Nov 12 at 13:41
<?= appwidgetsAddNewURL::widget() ?>
– Roboto6_1on
Nov 12 at 13:51
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I would like to have a form available on every page in my web app. So I created a widget with a form.
class AddNewURL extends Widget
public $model;
public function init()
parent::init();
if ($this->model === null)
$this->model = new ProductUrlForm();
public function run()
return $this->render('addNewURLForm', [
'model' => $this->model,
]);
and widget view
<div class="modal fade bs-example-modal-lg" id="newURL" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<?php Pjax::begin(); ?>
<?php $form = ActiveForm::begin([
'action' => Url::to(['site/new-url']),
'options' => ['data' => ['pjax' => true]],
]) ?>
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span>
</button>
<h4 class="modal-title" id="myModalLabel">Add new URL</h4>
</div>
<div class="modal-body">
<div class="row">
<div class="col-md-12">
<?= $form->field($model, 'url', ['errorOptions' => ['class' => 'help-block', 'encode' => false]])
->textInput(['maxlength' => false])
?>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Add</button>
</div>
<?php ActiveForm::end() ?>
<?php Pjax::end(); ?>
</div>
</div>
</div>
and this how I use this widget in my layouts/main.php
<?= appwidgetsAddNewURL::widget() ?>
in my site/new-url action I have
public function actionNewUrl()
$model = new ProductUrlForm();
$model->status = STATUS_NEW;
if ($model->load(Yii::$app->request->post()) && $model->save())
//return success message
return $this->render('index', [
'model' => $model,
]);
and now when I submit form data goes to my action, the form is reset and that's all. How to show validation error or success message using pjax? In my model I have few custom validation rules.
Maybe there is better solution to use one form on all pages?
forms yii2 widget pjax
I would like to have a form available on every page in my web app. So I created a widget with a form.
class AddNewURL extends Widget
public $model;
public function init()
parent::init();
if ($this->model === null)
$this->model = new ProductUrlForm();
public function run()
return $this->render('addNewURLForm', [
'model' => $this->model,
]);
and widget view
<div class="modal fade bs-example-modal-lg" id="newURL" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
<?php Pjax::begin(); ?>
<?php $form = ActiveForm::begin([
'action' => Url::to(['site/new-url']),
'options' => ['data' => ['pjax' => true]],
]) ?>
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span>
</button>
<h4 class="modal-title" id="myModalLabel">Add new URL</h4>
</div>
<div class="modal-body">
<div class="row">
<div class="col-md-12">
<?= $form->field($model, 'url', ['errorOptions' => ['class' => 'help-block', 'encode' => false]])
->textInput(['maxlength' => false])
?>
</div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
<button type="submit" class="btn btn-primary">Add</button>
</div>
<?php ActiveForm::end() ?>
<?php Pjax::end(); ?>
</div>
</div>
</div>
and this how I use this widget in my layouts/main.php
<?= appwidgetsAddNewURL::widget() ?>
in my site/new-url action I have
public function actionNewUrl()
$model = new ProductUrlForm();
$model->status = STATUS_NEW;
if ($model->load(Yii::$app->request->post()) && $model->save())
//return success message
return $this->render('index', [
'model' => $model,
]);
and now when I submit form data goes to my action, the form is reset and that's all. How to show validation error or success message using pjax? In my model I have few custom validation rules.
Maybe there is better solution to use one form on all pages?
forms yii2 widget pjax
forms yii2 widget pjax
edited Nov 12 at 13:54
asked Nov 12 at 0:19
Roboto6_1on
100112
100112
how and where are you calling this widget? add to your question.
– Muhammad Omer Aslam
Nov 12 at 13:41
<?= appwidgetsAddNewURL::widget() ?>
– Roboto6_1on
Nov 12 at 13:51
add a comment |
how and where are you calling this widget? add to your question.
– Muhammad Omer Aslam
Nov 12 at 13:41
<?= appwidgetsAddNewURL::widget() ?>
– Roboto6_1on
Nov 12 at 13:51
how and where are you calling this widget? add to your question.
– Muhammad Omer Aslam
Nov 12 at 13:41
how and where are you calling this widget? add to your question.
– Muhammad Omer Aslam
Nov 12 at 13:41
<?= appwidgetsAddNewURL::widget() ?>
– Roboto6_1on
Nov 12 at 13:51
<?= appwidgetsAddNewURL::widget() ?>
– Roboto6_1on
Nov 12 at 13:51
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Action actionNewUrl()
must render view addNewURLForm
so Pjax can replace old form with new html recieved from the action.
It is better to place your widget, controller and form model to separate module.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254568%2fvalidation-form-in-wiget%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Action actionNewUrl()
must render view addNewURLForm
so Pjax can replace old form with new html recieved from the action.
It is better to place your widget, controller and form model to separate module.
add a comment |
up vote
1
down vote
accepted
Action actionNewUrl()
must render view addNewURLForm
so Pjax can replace old form with new html recieved from the action.
It is better to place your widget, controller and form model to separate module.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Action actionNewUrl()
must render view addNewURLForm
so Pjax can replace old form with new html recieved from the action.
It is better to place your widget, controller and form model to separate module.
Action actionNewUrl()
must render view addNewURLForm
so Pjax can replace old form with new html recieved from the action.
It is better to place your widget, controller and form model to separate module.
answered Nov 13 at 5:27
Anton Rybalko
672816
672816
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53254568%2fvalidation-form-in-wiget%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
At FXoscaP0fNWc5yO49,RwOOfnBLE8l5GsaVWIw,Gq9CztEEP85tGt8f2bg WKn6SvvruVCIBKubg 39bm
how and where are you calling this widget? add to your question.
– Muhammad Omer Aslam
Nov 12 at 13:41
<?= appwidgetsAddNewURL::widget() ?>
– Roboto6_1on
Nov 12 at 13:51