Android WebView doesn't load url after returning to WebView page/activity
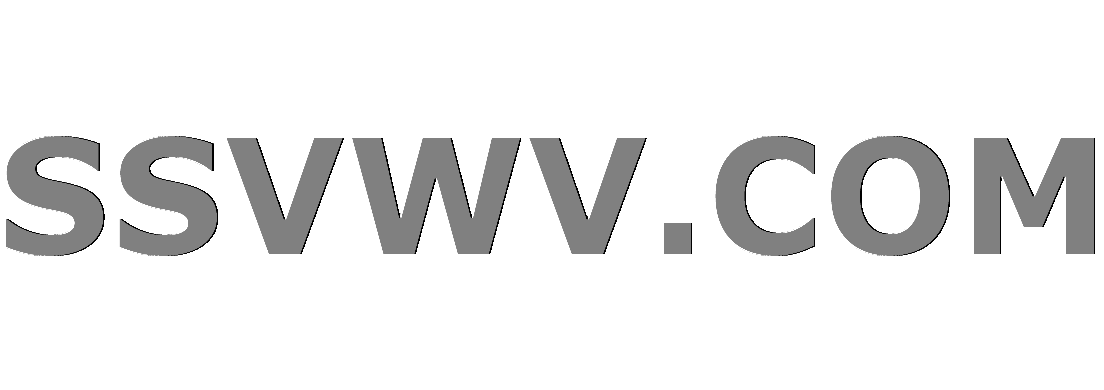
Multi tool use
Depending on WiFi connection (whether it's "on" or "off"), I want to display one of the two views/activities: No Connection or WebView with URL. I'm using BroadcastReceiver
to check the connection.
Everything seems to work fine when the app loads, but when I toggle WiFi "off" and then "on" again (whenever I leave WebView and then come back), WebView URL doesn't load (get Webpage not available message). No Connection view works always, so I'm assuming there is something with WebView. And also, WebView works if I add 5 second delay and then do loadUrl
again. I can also see that WiFi status is detected properly.
I would appreciate any input. I've googled it, but all I can find is basic WebView examples. I'm very new to Android, so maybe I'm missing something basic. Thanks in advance for your help!
```
class WebViewActivity : AppCompatActivity()
private lateinit var wifiManager : WifiManager
private lateinit var intentMain: Intent
private lateinit var webView: WebView
override fun onCreate(savedInstanceState: Bundle?)
Log.d("WIFI_TEST", "WV: onCreate")
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_web_view)
wifiManager = applicationContext.getSystemService(Context.WIFI_SERVICE) as WifiManager
intentMain = Intent(this, MainActivity::class.java)
webView = findViewById(R.id.webview)
webView.settings.javaScriptEnabled = true
webview.settings.domStorageEnabled = true
webView.webViewClient = WebViewClient()
webView.loadUrl("https://google.com")
override fun onStart()
super.onStart()
val intentFilter = IntentFilter(WifiManager.WIFI_STATE_CHANGED_ACTION)
registerReceiver(wifiStateReceiver, intentFilter)
override fun onStop()
super.onStop()
webView.destroy()
unregisterReceiver(wifiStateReceiver)
override fun onBackPressed()
if (webView.canGoBack())
webView.goBack()
else
super.onBackPressed()
private val wifiStateReceiver = object : BroadcastReceiver()
override fun onReceive(context: Context, intent: Intent)
val wifiStateExtra = intent.getIntExtra(
WifiManager.EXTRA_WIFI_STATE,
WifiManager.WIFI_STATE_UNKNOWN
)
when (wifiStateExtra)
WifiManager.WIFI_STATE_DISABLED ->
Log.d("WIFI_TEST", "WV: OFF")
startActivity(intentMain)
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
```

add a comment |
Depending on WiFi connection (whether it's "on" or "off"), I want to display one of the two views/activities: No Connection or WebView with URL. I'm using BroadcastReceiver
to check the connection.
Everything seems to work fine when the app loads, but when I toggle WiFi "off" and then "on" again (whenever I leave WebView and then come back), WebView URL doesn't load (get Webpage not available message). No Connection view works always, so I'm assuming there is something with WebView. And also, WebView works if I add 5 second delay and then do loadUrl
again. I can also see that WiFi status is detected properly.
I would appreciate any input. I've googled it, but all I can find is basic WebView examples. I'm very new to Android, so maybe I'm missing something basic. Thanks in advance for your help!
```
class WebViewActivity : AppCompatActivity()
private lateinit var wifiManager : WifiManager
private lateinit var intentMain: Intent
private lateinit var webView: WebView
override fun onCreate(savedInstanceState: Bundle?)
Log.d("WIFI_TEST", "WV: onCreate")
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_web_view)
wifiManager = applicationContext.getSystemService(Context.WIFI_SERVICE) as WifiManager
intentMain = Intent(this, MainActivity::class.java)
webView = findViewById(R.id.webview)
webView.settings.javaScriptEnabled = true
webview.settings.domStorageEnabled = true
webView.webViewClient = WebViewClient()
webView.loadUrl("https://google.com")
override fun onStart()
super.onStart()
val intentFilter = IntentFilter(WifiManager.WIFI_STATE_CHANGED_ACTION)
registerReceiver(wifiStateReceiver, intentFilter)
override fun onStop()
super.onStop()
webView.destroy()
unregisterReceiver(wifiStateReceiver)
override fun onBackPressed()
if (webView.canGoBack())
webView.goBack()
else
super.onBackPressed()
private val wifiStateReceiver = object : BroadcastReceiver()
override fun onReceive(context: Context, intent: Intent)
val wifiStateExtra = intent.getIntExtra(
WifiManager.EXTRA_WIFI_STATE,
WifiManager.WIFI_STATE_UNKNOWN
)
when (wifiStateExtra)
WifiManager.WIFI_STATE_DISABLED ->
Log.d("WIFI_TEST", "WV: OFF")
startActivity(intentMain)
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
```

add a comment |
Depending on WiFi connection (whether it's "on" or "off"), I want to display one of the two views/activities: No Connection or WebView with URL. I'm using BroadcastReceiver
to check the connection.
Everything seems to work fine when the app loads, but when I toggle WiFi "off" and then "on" again (whenever I leave WebView and then come back), WebView URL doesn't load (get Webpage not available message). No Connection view works always, so I'm assuming there is something with WebView. And also, WebView works if I add 5 second delay and then do loadUrl
again. I can also see that WiFi status is detected properly.
I would appreciate any input. I've googled it, but all I can find is basic WebView examples. I'm very new to Android, so maybe I'm missing something basic. Thanks in advance for your help!
```
class WebViewActivity : AppCompatActivity()
private lateinit var wifiManager : WifiManager
private lateinit var intentMain: Intent
private lateinit var webView: WebView
override fun onCreate(savedInstanceState: Bundle?)
Log.d("WIFI_TEST", "WV: onCreate")
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_web_view)
wifiManager = applicationContext.getSystemService(Context.WIFI_SERVICE) as WifiManager
intentMain = Intent(this, MainActivity::class.java)
webView = findViewById(R.id.webview)
webView.settings.javaScriptEnabled = true
webview.settings.domStorageEnabled = true
webView.webViewClient = WebViewClient()
webView.loadUrl("https://google.com")
override fun onStart()
super.onStart()
val intentFilter = IntentFilter(WifiManager.WIFI_STATE_CHANGED_ACTION)
registerReceiver(wifiStateReceiver, intentFilter)
override fun onStop()
super.onStop()
webView.destroy()
unregisterReceiver(wifiStateReceiver)
override fun onBackPressed()
if (webView.canGoBack())
webView.goBack()
else
super.onBackPressed()
private val wifiStateReceiver = object : BroadcastReceiver()
override fun onReceive(context: Context, intent: Intent)
val wifiStateExtra = intent.getIntExtra(
WifiManager.EXTRA_WIFI_STATE,
WifiManager.WIFI_STATE_UNKNOWN
)
when (wifiStateExtra)
WifiManager.WIFI_STATE_DISABLED ->
Log.d("WIFI_TEST", "WV: OFF")
startActivity(intentMain)
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
```

Depending on WiFi connection (whether it's "on" or "off"), I want to display one of the two views/activities: No Connection or WebView with URL. I'm using BroadcastReceiver
to check the connection.
Everything seems to work fine when the app loads, but when I toggle WiFi "off" and then "on" again (whenever I leave WebView and then come back), WebView URL doesn't load (get Webpage not available message). No Connection view works always, so I'm assuming there is something with WebView. And also, WebView works if I add 5 second delay and then do loadUrl
again. I can also see that WiFi status is detected properly.
I would appreciate any input. I've googled it, but all I can find is basic WebView examples. I'm very new to Android, so maybe I'm missing something basic. Thanks in advance for your help!
```
class WebViewActivity : AppCompatActivity()
private lateinit var wifiManager : WifiManager
private lateinit var intentMain: Intent
private lateinit var webView: WebView
override fun onCreate(savedInstanceState: Bundle?)
Log.d("WIFI_TEST", "WV: onCreate")
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_web_view)
wifiManager = applicationContext.getSystemService(Context.WIFI_SERVICE) as WifiManager
intentMain = Intent(this, MainActivity::class.java)
webView = findViewById(R.id.webview)
webView.settings.javaScriptEnabled = true
webview.settings.domStorageEnabled = true
webView.webViewClient = WebViewClient()
webView.loadUrl("https://google.com")
override fun onStart()
super.onStart()
val intentFilter = IntentFilter(WifiManager.WIFI_STATE_CHANGED_ACTION)
registerReceiver(wifiStateReceiver, intentFilter)
override fun onStop()
super.onStop()
webView.destroy()
unregisterReceiver(wifiStateReceiver)
override fun onBackPressed()
if (webView.canGoBack())
webView.goBack()
else
super.onBackPressed()
private val wifiStateReceiver = object : BroadcastReceiver()
override fun onReceive(context: Context, intent: Intent)
val wifiStateExtra = intent.getIntExtra(
WifiManager.EXTRA_WIFI_STATE,
WifiManager.WIFI_STATE_UNKNOWN
)
when (wifiStateExtra)
WifiManager.WIFI_STATE_DISABLED ->
Log.d("WIFI_TEST", "WV: OFF")
startActivity(intentMain)
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
```


asked Nov 15 '18 at 22:40
quietbitsquietbits
14319
14319
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Remember that when the Wifi is enabled again probably is available but you have to wait some milliseconds for connectivity.
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
isAvailable(): Indicates whether network connectivity is possible. A
network is unavailable when a persistent or semi-persistent condition
prevents the possibility of connecting to that network.
isConnected(): Indicates whether network connectivity exists and it is
possible to establish connections and pass data.
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328883%2fandroid-webview-doesnt-load-url-after-returning-to-webview-page-activity%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Remember that when the Wifi is enabled again probably is available but you have to wait some milliseconds for connectivity.
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
isAvailable(): Indicates whether network connectivity is possible. A
network is unavailable when a persistent or semi-persistent condition
prevents the possibility of connecting to that network.
isConnected(): Indicates whether network connectivity exists and it is
possible to establish connections and pass data.
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
add a comment |
Remember that when the Wifi is enabled again probably is available but you have to wait some milliseconds for connectivity.
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
isAvailable(): Indicates whether network connectivity is possible. A
network is unavailable when a persistent or semi-persistent condition
prevents the possibility of connecting to that network.
isConnected(): Indicates whether network connectivity exists and it is
possible to establish connections and pass data.
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
add a comment |
Remember that when the Wifi is enabled again probably is available but you have to wait some milliseconds for connectivity.
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
isAvailable(): Indicates whether network connectivity is possible. A
network is unavailable when a persistent or semi-persistent condition
prevents the possibility of connecting to that network.
isConnected(): Indicates whether network connectivity exists and it is
possible to establish connections and pass data.
Remember that when the Wifi is enabled again probably is available but you have to wait some milliseconds for connectivity.
WifiManager.WIFI_STATE_ENABLED ->
Log.d("WIFI_TEST", "WV: ON")
// Works with delay with at least 5 sec
// Why?
Timer().schedule(5000)
Log.d("WIFI_TEST", "WV: delay")
webView.post(Runnable
webView.loadUrl("https://google.com")
)
isAvailable(): Indicates whether network connectivity is possible. A
network is unavailable when a persistent or semi-persistent condition
prevents the possibility of connecting to that network.
isConnected(): Indicates whether network connectivity exists and it is
possible to establish connections and pass data.
answered Nov 16 '18 at 0:55
ElenasysElenasys
95k16248215
95k16248215
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
add a comment |
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
Thanks for your quick response! I see what you mean, will try and update with progress.
– quietbits
Nov 16 '18 at 15:01
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328883%2fandroid-webview-doesnt-load-url-after-returning-to-webview-page-activity%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sm1gC OCnWd M ca5hkcE,qXc7KWKILJdQJ4PoYTONf i