How do I display a SQLite table in a QTableView
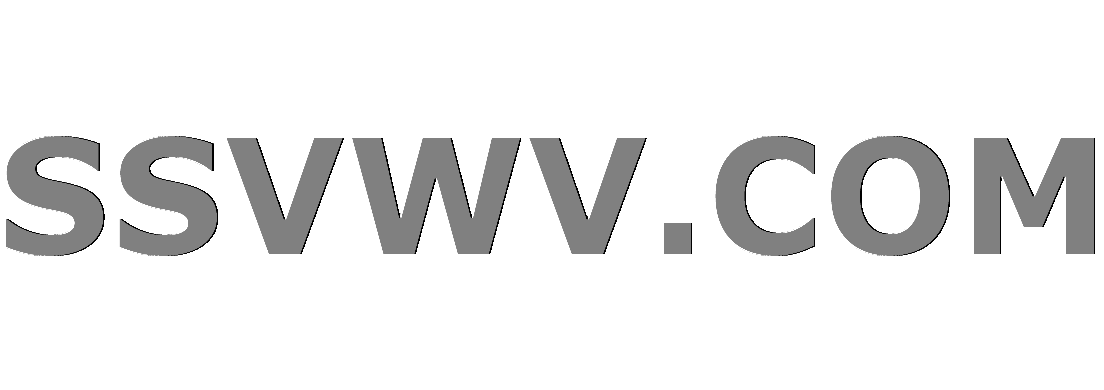
Multi tool use
I am trying to connect to a local database and show a table in QTableView. I am currently getting a connection to my database but whenever I try to append my query to the QTableView box i am getting QSqlError("", "Unable to find table projects", "")
. When I run SELECT * FROM projects
in DB browser for SQLite, the entry I have in that table shows up. Here is my mainwindow.h:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "purchaseorder.h"
#include <QtSql>
namespace Ui
class MainWindow;
class MainWindow : public QMainWindow
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
PurchaseOrder *newpo = new PurchaseOrder();
QSqlDatabase db;
bool openDB()
db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("projectmanager.db");
if(!db.open())
qDebug()<<"Error opening database" << db.lastError();
return false;
else
qDebug()<<"Connection Established.";
return true;
void closeDB()
db.close();
//db.removeDatabase(QSqlDatabase::defaultConnection);
private slots:
void on_actionProject_2_triggered();
private:
Ui::MainWindow *ui;
PurchaseOrder purchaseorder;
;
#endif // MAINWINDOW_H
Here is a snippet of my mainwindow.cpp:
#include "mainwindow.h"
#include <QDebug>
#include <QSqlQueryModel>
#include <QtSql>
void MainWindow::on_actionProject_2_triggered() // Load PROJECT table
QSqlTableModel* modal = new QSqlTableModel();
MainWindow conn;
conn.openDB();
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
qDebug()<<modal->lastError();
conn.close();
I believe everything is working up until. modal->setTable("projects");
Also I have my projectmanager.db
file stored in /db/projectmanager.db
but when I put that in my db path it does not connect but it will connect the way I have it? Thank you for any help, all is appreciated.
Desc. of projects:
CREATE TABLE `projects` ( `project_id` INTEGER PRIMARY KEY AUTOINCREMENT,
`project_name` TEXT,
`client` INTEGER,
`lead_employee` INTEGER,
`description` TEXT,
`start_date` TEXT,
`deadline` TEXT,
`status` INTEGER )
c++ qt sqlite qtableview qtsql
|
show 4 more comments
I am trying to connect to a local database and show a table in QTableView. I am currently getting a connection to my database but whenever I try to append my query to the QTableView box i am getting QSqlError("", "Unable to find table projects", "")
. When I run SELECT * FROM projects
in DB browser for SQLite, the entry I have in that table shows up. Here is my mainwindow.h:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "purchaseorder.h"
#include <QtSql>
namespace Ui
class MainWindow;
class MainWindow : public QMainWindow
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
PurchaseOrder *newpo = new PurchaseOrder();
QSqlDatabase db;
bool openDB()
db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("projectmanager.db");
if(!db.open())
qDebug()<<"Error opening database" << db.lastError();
return false;
else
qDebug()<<"Connection Established.";
return true;
void closeDB()
db.close();
//db.removeDatabase(QSqlDatabase::defaultConnection);
private slots:
void on_actionProject_2_triggered();
private:
Ui::MainWindow *ui;
PurchaseOrder purchaseorder;
;
#endif // MAINWINDOW_H
Here is a snippet of my mainwindow.cpp:
#include "mainwindow.h"
#include <QDebug>
#include <QSqlQueryModel>
#include <QtSql>
void MainWindow::on_actionProject_2_triggered() // Load PROJECT table
QSqlTableModel* modal = new QSqlTableModel();
MainWindow conn;
conn.openDB();
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
qDebug()<<modal->lastError();
conn.close();
I believe everything is working up until. modal->setTable("projects");
Also I have my projectmanager.db
file stored in /db/projectmanager.db
but when I put that in my db path it does not connect but it will connect the way I have it? Thank you for any help, all is appreciated.
Desc. of projects:
CREATE TABLE `projects` ( `project_id` INTEGER PRIMARY KEY AUTOINCREMENT,
`project_name` TEXT,
`client` INTEGER,
`lead_employee` INTEGER,
`description` TEXT,
`start_date` TEXT,
`deadline` TEXT,
`status` INTEGER )
c++ qt sqlite qtableview qtsql
QSqlDatabase db;
It is highly recommended thatQSqlDatabase
types not be used as member variables. Please provide a minimal, complete, and verifiable example. Right now, your question is kinda close but not yet complete. (e.g. sample database data?)
– TrebledJ
Nov 16 '18 at 3:42
@TrebuchetMS Thank you for the suggestion I have added the table that I am trying to acquire. Please let me know if there is more that I can provide.
– Jordan
Nov 16 '18 at 3:48
Is/db/projectmanager.db
the full file path? If not, replace it with the full path. (If the best you could work with is a relative path, consider using QDir.)
– TrebledJ
Nov 16 '18 at 3:54
@TrebuchetMS The full path isC:/Users/jorda/Documents/divisionrepo/tdproject/db/projectmanager.db
I should have mentioned in the problem that I had tried this as well with the same result (Connection Established, Cannot find table projects).
– Jordan
Nov 16 '18 at 3:57
@TrebuchetMS if I delete the .db file from that path it still runs as well with "Connection established.".
– Jordan
Nov 16 '18 at 3:59
|
show 4 more comments
I am trying to connect to a local database and show a table in QTableView. I am currently getting a connection to my database but whenever I try to append my query to the QTableView box i am getting QSqlError("", "Unable to find table projects", "")
. When I run SELECT * FROM projects
in DB browser for SQLite, the entry I have in that table shows up. Here is my mainwindow.h:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "purchaseorder.h"
#include <QtSql>
namespace Ui
class MainWindow;
class MainWindow : public QMainWindow
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
PurchaseOrder *newpo = new PurchaseOrder();
QSqlDatabase db;
bool openDB()
db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("projectmanager.db");
if(!db.open())
qDebug()<<"Error opening database" << db.lastError();
return false;
else
qDebug()<<"Connection Established.";
return true;
void closeDB()
db.close();
//db.removeDatabase(QSqlDatabase::defaultConnection);
private slots:
void on_actionProject_2_triggered();
private:
Ui::MainWindow *ui;
PurchaseOrder purchaseorder;
;
#endif // MAINWINDOW_H
Here is a snippet of my mainwindow.cpp:
#include "mainwindow.h"
#include <QDebug>
#include <QSqlQueryModel>
#include <QtSql>
void MainWindow::on_actionProject_2_triggered() // Load PROJECT table
QSqlTableModel* modal = new QSqlTableModel();
MainWindow conn;
conn.openDB();
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
qDebug()<<modal->lastError();
conn.close();
I believe everything is working up until. modal->setTable("projects");
Also I have my projectmanager.db
file stored in /db/projectmanager.db
but when I put that in my db path it does not connect but it will connect the way I have it? Thank you for any help, all is appreciated.
Desc. of projects:
CREATE TABLE `projects` ( `project_id` INTEGER PRIMARY KEY AUTOINCREMENT,
`project_name` TEXT,
`client` INTEGER,
`lead_employee` INTEGER,
`description` TEXT,
`start_date` TEXT,
`deadline` TEXT,
`status` INTEGER )
c++ qt sqlite qtableview qtsql
I am trying to connect to a local database and show a table in QTableView. I am currently getting a connection to my database but whenever I try to append my query to the QTableView box i am getting QSqlError("", "Unable to find table projects", "")
. When I run SELECT * FROM projects
in DB browser for SQLite, the entry I have in that table shows up. Here is my mainwindow.h:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "purchaseorder.h"
#include <QtSql>
namespace Ui
class MainWindow;
class MainWindow : public QMainWindow
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
PurchaseOrder *newpo = new PurchaseOrder();
QSqlDatabase db;
bool openDB()
db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("projectmanager.db");
if(!db.open())
qDebug()<<"Error opening database" << db.lastError();
return false;
else
qDebug()<<"Connection Established.";
return true;
void closeDB()
db.close();
//db.removeDatabase(QSqlDatabase::defaultConnection);
private slots:
void on_actionProject_2_triggered();
private:
Ui::MainWindow *ui;
PurchaseOrder purchaseorder;
;
#endif // MAINWINDOW_H
Here is a snippet of my mainwindow.cpp:
#include "mainwindow.h"
#include <QDebug>
#include <QSqlQueryModel>
#include <QtSql>
void MainWindow::on_actionProject_2_triggered() // Load PROJECT table
QSqlTableModel* modal = new QSqlTableModel();
MainWindow conn;
conn.openDB();
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
qDebug()<<modal->lastError();
conn.close();
I believe everything is working up until. modal->setTable("projects");
Also I have my projectmanager.db
file stored in /db/projectmanager.db
but when I put that in my db path it does not connect but it will connect the way I have it? Thank you for any help, all is appreciated.
Desc. of projects:
CREATE TABLE `projects` ( `project_id` INTEGER PRIMARY KEY AUTOINCREMENT,
`project_name` TEXT,
`client` INTEGER,
`lead_employee` INTEGER,
`description` TEXT,
`start_date` TEXT,
`deadline` TEXT,
`status` INTEGER )
c++ qt sqlite qtableview qtsql
c++ qt sqlite qtableview qtsql
edited Nov 16 '18 at 3:47
Jordan
asked Nov 16 '18 at 3:32


JordanJordan
175
175
QSqlDatabase db;
It is highly recommended thatQSqlDatabase
types not be used as member variables. Please provide a minimal, complete, and verifiable example. Right now, your question is kinda close but not yet complete. (e.g. sample database data?)
– TrebledJ
Nov 16 '18 at 3:42
@TrebuchetMS Thank you for the suggestion I have added the table that I am trying to acquire. Please let me know if there is more that I can provide.
– Jordan
Nov 16 '18 at 3:48
Is/db/projectmanager.db
the full file path? If not, replace it with the full path. (If the best you could work with is a relative path, consider using QDir.)
– TrebledJ
Nov 16 '18 at 3:54
@TrebuchetMS The full path isC:/Users/jorda/Documents/divisionrepo/tdproject/db/projectmanager.db
I should have mentioned in the problem that I had tried this as well with the same result (Connection Established, Cannot find table projects).
– Jordan
Nov 16 '18 at 3:57
@TrebuchetMS if I delete the .db file from that path it still runs as well with "Connection established.".
– Jordan
Nov 16 '18 at 3:59
|
show 4 more comments
QSqlDatabase db;
It is highly recommended thatQSqlDatabase
types not be used as member variables. Please provide a minimal, complete, and verifiable example. Right now, your question is kinda close but not yet complete. (e.g. sample database data?)
– TrebledJ
Nov 16 '18 at 3:42
@TrebuchetMS Thank you for the suggestion I have added the table that I am trying to acquire. Please let me know if there is more that I can provide.
– Jordan
Nov 16 '18 at 3:48
Is/db/projectmanager.db
the full file path? If not, replace it with the full path. (If the best you could work with is a relative path, consider using QDir.)
– TrebledJ
Nov 16 '18 at 3:54
@TrebuchetMS The full path isC:/Users/jorda/Documents/divisionrepo/tdproject/db/projectmanager.db
I should have mentioned in the problem that I had tried this as well with the same result (Connection Established, Cannot find table projects).
– Jordan
Nov 16 '18 at 3:57
@TrebuchetMS if I delete the .db file from that path it still runs as well with "Connection established.".
– Jordan
Nov 16 '18 at 3:59
QSqlDatabase db;
It is highly recommended that QSqlDatabase
types not be used as member variables. Please provide a minimal, complete, and verifiable example. Right now, your question is kinda close but not yet complete. (e.g. sample database data?)– TrebledJ
Nov 16 '18 at 3:42
QSqlDatabase db;
It is highly recommended that QSqlDatabase
types not be used as member variables. Please provide a minimal, complete, and verifiable example. Right now, your question is kinda close but not yet complete. (e.g. sample database data?)– TrebledJ
Nov 16 '18 at 3:42
@TrebuchetMS Thank you for the suggestion I have added the table that I am trying to acquire. Please let me know if there is more that I can provide.
– Jordan
Nov 16 '18 at 3:48
@TrebuchetMS Thank you for the suggestion I have added the table that I am trying to acquire. Please let me know if there is more that I can provide.
– Jordan
Nov 16 '18 at 3:48
Is
/db/projectmanager.db
the full file path? If not, replace it with the full path. (If the best you could work with is a relative path, consider using QDir.)– TrebledJ
Nov 16 '18 at 3:54
Is
/db/projectmanager.db
the full file path? If not, replace it with the full path. (If the best you could work with is a relative path, consider using QDir.)– TrebledJ
Nov 16 '18 at 3:54
@TrebuchetMS The full path is
C:/Users/jorda/Documents/divisionrepo/tdproject/db/projectmanager.db
I should have mentioned in the problem that I had tried this as well with the same result (Connection Established, Cannot find table projects).– Jordan
Nov 16 '18 at 3:57
@TrebuchetMS The full path is
C:/Users/jorda/Documents/divisionrepo/tdproject/db/projectmanager.db
I should have mentioned in the problem that I had tried this as well with the same result (Connection Established, Cannot find table projects).– Jordan
Nov 16 '18 at 3:57
@TrebuchetMS if I delete the .db file from that path it still runs as well with "Connection established.".
– Jordan
Nov 16 '18 at 3:59
@TrebuchetMS if I delete the .db file from that path it still runs as well with "Connection established.".
– Jordan
Nov 16 '18 at 3:59
|
show 4 more comments
1 Answer
1
active
oldest
votes
You have to open the connection before creating the model, on the other hand it is not necessary to create another MainWindow, so the solution is:
void MainWindow::on_actionProject_2_triggered()
openDB();
QSqlTableModel *modal = new QSqlTableModel;
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
closeDB();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331018%2fhow-do-i-display-a-sqlite-table-in-a-qtableview%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have to open the connection before creating the model, on the other hand it is not necessary to create another MainWindow, so the solution is:
void MainWindow::on_actionProject_2_triggered()
openDB();
QSqlTableModel *modal = new QSqlTableModel;
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
closeDB();
add a comment |
You have to open the connection before creating the model, on the other hand it is not necessary to create another MainWindow, so the solution is:
void MainWindow::on_actionProject_2_triggered()
openDB();
QSqlTableModel *modal = new QSqlTableModel;
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
closeDB();
add a comment |
You have to open the connection before creating the model, on the other hand it is not necessary to create another MainWindow, so the solution is:
void MainWindow::on_actionProject_2_triggered()
openDB();
QSqlTableModel *modal = new QSqlTableModel;
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
closeDB();
You have to open the connection before creating the model, on the other hand it is not necessary to create another MainWindow, so the solution is:
void MainWindow::on_actionProject_2_triggered()
openDB();
QSqlTableModel *modal = new QSqlTableModel;
modal->setTable("projects");
modal->select();
ui->tableView->setModel(modal);
closeDB();
answered Nov 16 '18 at 4:20


eyllanesceyllanesc
83.8k103562
83.8k103562
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331018%2fhow-do-i-display-a-sqlite-table-in-a-qtableview%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oDsFgn,tru Q0m4p,C tnWISCLRCLXu,Fz,19A,7,vA1EOwFrH,OBu,d
QSqlDatabase db;
It is highly recommended thatQSqlDatabase
types not be used as member variables. Please provide a minimal, complete, and verifiable example. Right now, your question is kinda close but not yet complete. (e.g. sample database data?)– TrebledJ
Nov 16 '18 at 3:42
@TrebuchetMS Thank you for the suggestion I have added the table that I am trying to acquire. Please let me know if there is more that I can provide.
– Jordan
Nov 16 '18 at 3:48
Is
/db/projectmanager.db
the full file path? If not, replace it with the full path. (If the best you could work with is a relative path, consider using QDir.)– TrebledJ
Nov 16 '18 at 3:54
@TrebuchetMS The full path is
C:/Users/jorda/Documents/divisionrepo/tdproject/db/projectmanager.db
I should have mentioned in the problem that I had tried this as well with the same result (Connection Established, Cannot find table projects).– Jordan
Nov 16 '18 at 3:57
@TrebuchetMS if I delete the .db file from that path it still runs as well with "Connection established.".
– Jordan
Nov 16 '18 at 3:59