Insertion Sort Python to create a list with two dependent variables
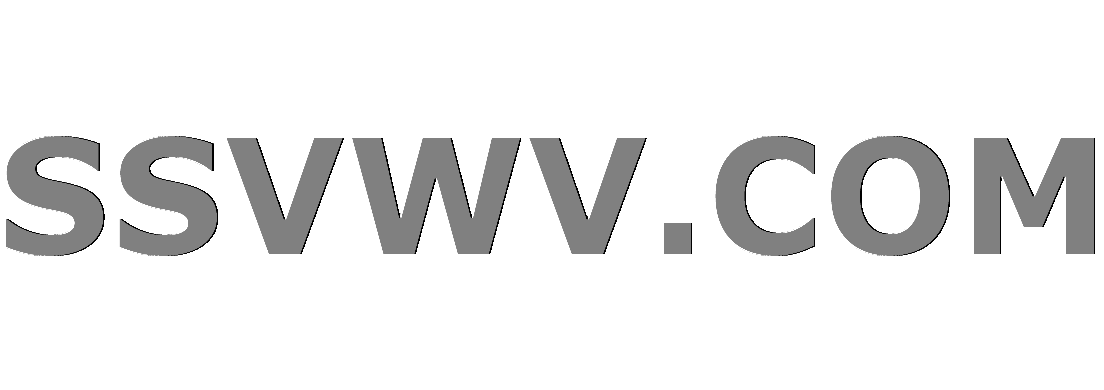
Multi tool use
First python program, and first question on Stack Overflow. I'm definitely punching out of my weight class with what I'm trying to do, but I've always heard that one of the best motivations for learning to program is wanting to solve a problem, and that turned out to be the case for me.
I'm trying to get Snips to go to my private calendar's RSS feed, and parse my upcoming appointments, and use TTS to tell me what I've got going that day. I've made a lot of progress (at least as I'm seeing it) and at this point, I can run my program and it successfully goes to the RSS feed using feedparser.py and selects all of the events on the current day (learning about dates and time conversions in Python took me quite a bit of time!), and sends a MQTT message back to Snips, which used PicoTTS to read them to me. The issue I'm having is that the Webcalendar RSS feed orders the event entries by which is formatted as GMT time. I'm not living in the GMT timezone, so I've learned enough Python to to correctly format GMT time to my timezone (thanks to StackOverflow and a lot of reading!) so I'm able to correctly select events that are scheduled for the day. As an example, here's the RSS feed for yesterday, showing two events, both of which occur on 14 Nov 2018 when the correct timezone is applied (PST).
<?xml version="1.0" encoding="iso-8859-1"?>
<rss version="2.0" xml:lang="en-us">
<channel>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<description><![CDATA[WebCalendar]]></description>
<language>en-us</language>
<generator>:"http://www.k5n.us/webcalendar.php?v=v1.2.7"</generator>
<image>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<url>http://www.k5n.us/k5n_small.gif</url>
</image>
<item>
<title><![CDATA[Test Appointment 6]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 6 oclock]]></description>
<pubDate>Thu, 15 Nov 2018 02:00:00 GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
<item>
<title><![CDATA[Test Appointment 2]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 8]]></description>
<pubDate>Wed, 14 Nov 2018 22:00:00 GMT GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
So, here's my issue. Because of how Webcalendar presents events (ordered by by GMT date/time) in the RSS feed and how I'm looping through all of the entries from first to last, I'm getting events presented in the wrong order, e.g. Appointment 6 (which is a 6 o'clock pm PST time ) is entered into my list BEFORE Appointment 2 (which is at 2 o'clock PST time) and when the list is sent to Snips via MQTT, the events are read back to me out of order (not what I want!)
I think that I can use Python insertion sort to fix the issue, (but I'm open to other suggestions!) based on this Stack Overflow posting (How does Python insertion sort work?) but I'm not sure how I can keep title and pubDate from the RSS feed together when I'm using insertion sort to the list since I need the list to contain both entries, and my strings to make the TTS make sense since all of the examples for insertion sort I've found are shown using a it to sort a single variable.
Any help or direction would be very much appreciated!!
d = feedparser.parse('http://XX.XX.XX.XX/WebCalendar/rss.php?numdays=3&repeats=1')
global parsedListOfCalendarEntryTitles # Create a global variable:parsedListOfCalendarEntryTitles that will be used to hold the list of parsed RSS feed entry titles & Time
parsedListOfCalendarEntryTitles = # Define the global variable: parsedListOfCalendarEntryTitles as a list
global mqttFormattedListOfCalendarEntryTitles #Create a global variable: mqttFormattedListOfCalendarEntryTitles that will be used to hold the list of MQTT RSS feed entry titles & Time
mqttFormattedListOfCalendarEntryTitles = # Define the global variable: mqttFormattedListOfCalendarEntryTitles as a list
for entry in d.entries:
from_zone = tz.tzutc()
to_zone = tz.tzlocal()
date = entry.published
utc = datetime.strptime(date, '%a, %d %b %Y %H:%M:%S %Z')
utc = utc.replace(tzinfo=from_zone)
pacificTime = utc.astimezone(to_zone)
today = datetime.now()
if today.date() == pacificTime.date():
parsedListOfCalendarEntryTitles.append("You have " + entry.title + " at " + pacificTime.strftime("%I:%M %p"))
print(parsedListOfCalendarEntryTitles)
python snips
add a comment |
First python program, and first question on Stack Overflow. I'm definitely punching out of my weight class with what I'm trying to do, but I've always heard that one of the best motivations for learning to program is wanting to solve a problem, and that turned out to be the case for me.
I'm trying to get Snips to go to my private calendar's RSS feed, and parse my upcoming appointments, and use TTS to tell me what I've got going that day. I've made a lot of progress (at least as I'm seeing it) and at this point, I can run my program and it successfully goes to the RSS feed using feedparser.py and selects all of the events on the current day (learning about dates and time conversions in Python took me quite a bit of time!), and sends a MQTT message back to Snips, which used PicoTTS to read them to me. The issue I'm having is that the Webcalendar RSS feed orders the event entries by which is formatted as GMT time. I'm not living in the GMT timezone, so I've learned enough Python to to correctly format GMT time to my timezone (thanks to StackOverflow and a lot of reading!) so I'm able to correctly select events that are scheduled for the day. As an example, here's the RSS feed for yesterday, showing two events, both of which occur on 14 Nov 2018 when the correct timezone is applied (PST).
<?xml version="1.0" encoding="iso-8859-1"?>
<rss version="2.0" xml:lang="en-us">
<channel>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<description><![CDATA[WebCalendar]]></description>
<language>en-us</language>
<generator>:"http://www.k5n.us/webcalendar.php?v=v1.2.7"</generator>
<image>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<url>http://www.k5n.us/k5n_small.gif</url>
</image>
<item>
<title><![CDATA[Test Appointment 6]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 6 oclock]]></description>
<pubDate>Thu, 15 Nov 2018 02:00:00 GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
<item>
<title><![CDATA[Test Appointment 2]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 8]]></description>
<pubDate>Wed, 14 Nov 2018 22:00:00 GMT GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
So, here's my issue. Because of how Webcalendar presents events (ordered by by GMT date/time) in the RSS feed and how I'm looping through all of the entries from first to last, I'm getting events presented in the wrong order, e.g. Appointment 6 (which is a 6 o'clock pm PST time ) is entered into my list BEFORE Appointment 2 (which is at 2 o'clock PST time) and when the list is sent to Snips via MQTT, the events are read back to me out of order (not what I want!)
I think that I can use Python insertion sort to fix the issue, (but I'm open to other suggestions!) based on this Stack Overflow posting (How does Python insertion sort work?) but I'm not sure how I can keep title and pubDate from the RSS feed together when I'm using insertion sort to the list since I need the list to contain both entries, and my strings to make the TTS make sense since all of the examples for insertion sort I've found are shown using a it to sort a single variable.
Any help or direction would be very much appreciated!!
d = feedparser.parse('http://XX.XX.XX.XX/WebCalendar/rss.php?numdays=3&repeats=1')
global parsedListOfCalendarEntryTitles # Create a global variable:parsedListOfCalendarEntryTitles that will be used to hold the list of parsed RSS feed entry titles & Time
parsedListOfCalendarEntryTitles = # Define the global variable: parsedListOfCalendarEntryTitles as a list
global mqttFormattedListOfCalendarEntryTitles #Create a global variable: mqttFormattedListOfCalendarEntryTitles that will be used to hold the list of MQTT RSS feed entry titles & Time
mqttFormattedListOfCalendarEntryTitles = # Define the global variable: mqttFormattedListOfCalendarEntryTitles as a list
for entry in d.entries:
from_zone = tz.tzutc()
to_zone = tz.tzlocal()
date = entry.published
utc = datetime.strptime(date, '%a, %d %b %Y %H:%M:%S %Z')
utc = utc.replace(tzinfo=from_zone)
pacificTime = utc.astimezone(to_zone)
today = datetime.now()
if today.date() == pacificTime.date():
parsedListOfCalendarEntryTitles.append("You have " + entry.title + " at " + pacificTime.strftime("%I:%M %p"))
print(parsedListOfCalendarEntryTitles)
python snips
add a comment |
First python program, and first question on Stack Overflow. I'm definitely punching out of my weight class with what I'm trying to do, but I've always heard that one of the best motivations for learning to program is wanting to solve a problem, and that turned out to be the case for me.
I'm trying to get Snips to go to my private calendar's RSS feed, and parse my upcoming appointments, and use TTS to tell me what I've got going that day. I've made a lot of progress (at least as I'm seeing it) and at this point, I can run my program and it successfully goes to the RSS feed using feedparser.py and selects all of the events on the current day (learning about dates and time conversions in Python took me quite a bit of time!), and sends a MQTT message back to Snips, which used PicoTTS to read them to me. The issue I'm having is that the Webcalendar RSS feed orders the event entries by which is formatted as GMT time. I'm not living in the GMT timezone, so I've learned enough Python to to correctly format GMT time to my timezone (thanks to StackOverflow and a lot of reading!) so I'm able to correctly select events that are scheduled for the day. As an example, here's the RSS feed for yesterday, showing two events, both of which occur on 14 Nov 2018 when the correct timezone is applied (PST).
<?xml version="1.0" encoding="iso-8859-1"?>
<rss version="2.0" xml:lang="en-us">
<channel>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<description><![CDATA[WebCalendar]]></description>
<language>en-us</language>
<generator>:"http://www.k5n.us/webcalendar.php?v=v1.2.7"</generator>
<image>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<url>http://www.k5n.us/k5n_small.gif</url>
</image>
<item>
<title><![CDATA[Test Appointment 6]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 6 oclock]]></description>
<pubDate>Thu, 15 Nov 2018 02:00:00 GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
<item>
<title><![CDATA[Test Appointment 2]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 8]]></description>
<pubDate>Wed, 14 Nov 2018 22:00:00 GMT GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
So, here's my issue. Because of how Webcalendar presents events (ordered by by GMT date/time) in the RSS feed and how I'm looping through all of the entries from first to last, I'm getting events presented in the wrong order, e.g. Appointment 6 (which is a 6 o'clock pm PST time ) is entered into my list BEFORE Appointment 2 (which is at 2 o'clock PST time) and when the list is sent to Snips via MQTT, the events are read back to me out of order (not what I want!)
I think that I can use Python insertion sort to fix the issue, (but I'm open to other suggestions!) based on this Stack Overflow posting (How does Python insertion sort work?) but I'm not sure how I can keep title and pubDate from the RSS feed together when I'm using insertion sort to the list since I need the list to contain both entries, and my strings to make the TTS make sense since all of the examples for insertion sort I've found are shown using a it to sort a single variable.
Any help or direction would be very much appreciated!!
d = feedparser.parse('http://XX.XX.XX.XX/WebCalendar/rss.php?numdays=3&repeats=1')
global parsedListOfCalendarEntryTitles # Create a global variable:parsedListOfCalendarEntryTitles that will be used to hold the list of parsed RSS feed entry titles & Time
parsedListOfCalendarEntryTitles = # Define the global variable: parsedListOfCalendarEntryTitles as a list
global mqttFormattedListOfCalendarEntryTitles #Create a global variable: mqttFormattedListOfCalendarEntryTitles that will be used to hold the list of MQTT RSS feed entry titles & Time
mqttFormattedListOfCalendarEntryTitles = # Define the global variable: mqttFormattedListOfCalendarEntryTitles as a list
for entry in d.entries:
from_zone = tz.tzutc()
to_zone = tz.tzlocal()
date = entry.published
utc = datetime.strptime(date, '%a, %d %b %Y %H:%M:%S %Z')
utc = utc.replace(tzinfo=from_zone)
pacificTime = utc.astimezone(to_zone)
today = datetime.now()
if today.date() == pacificTime.date():
parsedListOfCalendarEntryTitles.append("You have " + entry.title + " at " + pacificTime.strftime("%I:%M %p"))
print(parsedListOfCalendarEntryTitles)
python snips
First python program, and first question on Stack Overflow. I'm definitely punching out of my weight class with what I'm trying to do, but I've always heard that one of the best motivations for learning to program is wanting to solve a problem, and that turned out to be the case for me.
I'm trying to get Snips to go to my private calendar's RSS feed, and parse my upcoming appointments, and use TTS to tell me what I've got going that day. I've made a lot of progress (at least as I'm seeing it) and at this point, I can run my program and it successfully goes to the RSS feed using feedparser.py and selects all of the events on the current day (learning about dates and time conversions in Python took me quite a bit of time!), and sends a MQTT message back to Snips, which used PicoTTS to read them to me. The issue I'm having is that the Webcalendar RSS feed orders the event entries by which is formatted as GMT time. I'm not living in the GMT timezone, so I've learned enough Python to to correctly format GMT time to my timezone (thanks to StackOverflow and a lot of reading!) so I'm able to correctly select events that are scheduled for the day. As an example, here's the RSS feed for yesterday, showing two events, both of which occur on 14 Nov 2018 when the correct timezone is applied (PST).
<?xml version="1.0" encoding="iso-8859-1"?>
<rss version="2.0" xml:lang="en-us">
<channel>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<description><![CDATA[WebCalendar]]></description>
<language>en-us</language>
<generator>:"http://www.k5n.us/webcalendar.php?v=v1.2.7"</generator>
<image>
<title><![CDATA[WebCalendar]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/</link>
<url>http://www.k5n.us/k5n_small.gif</url>
</image>
<item>
<title><![CDATA[Test Appointment 6]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 6 oclock]]></description>
<pubDate>Thu, 15 Nov 2018 02:00:00 GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=245&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
<item>
<title><![CDATA[Test Appointment 2]]></title>
<link>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</link>
<description><![CDATA[Test Appointment 8]]></description>
<pubDate>Wed, 14 Nov 2018 22:00:00 GMT GMT</pubDate>
<guid>http://XX.XX.XX.XX/WebCalendar/view_entry.php?id=246&friendly=1&rssuser=__public__&date=20181114</guid>
</item>
So, here's my issue. Because of how Webcalendar presents events (ordered by by GMT date/time) in the RSS feed and how I'm looping through all of the entries from first to last, I'm getting events presented in the wrong order, e.g. Appointment 6 (which is a 6 o'clock pm PST time ) is entered into my list BEFORE Appointment 2 (which is at 2 o'clock PST time) and when the list is sent to Snips via MQTT, the events are read back to me out of order (not what I want!)
I think that I can use Python insertion sort to fix the issue, (but I'm open to other suggestions!) based on this Stack Overflow posting (How does Python insertion sort work?) but I'm not sure how I can keep title and pubDate from the RSS feed together when I'm using insertion sort to the list since I need the list to contain both entries, and my strings to make the TTS make sense since all of the examples for insertion sort I've found are shown using a it to sort a single variable.
Any help or direction would be very much appreciated!!
d = feedparser.parse('http://XX.XX.XX.XX/WebCalendar/rss.php?numdays=3&repeats=1')
global parsedListOfCalendarEntryTitles # Create a global variable:parsedListOfCalendarEntryTitles that will be used to hold the list of parsed RSS feed entry titles & Time
parsedListOfCalendarEntryTitles = # Define the global variable: parsedListOfCalendarEntryTitles as a list
global mqttFormattedListOfCalendarEntryTitles #Create a global variable: mqttFormattedListOfCalendarEntryTitles that will be used to hold the list of MQTT RSS feed entry titles & Time
mqttFormattedListOfCalendarEntryTitles = # Define the global variable: mqttFormattedListOfCalendarEntryTitles as a list
for entry in d.entries:
from_zone = tz.tzutc()
to_zone = tz.tzlocal()
date = entry.published
utc = datetime.strptime(date, '%a, %d %b %Y %H:%M:%S %Z')
utc = utc.replace(tzinfo=from_zone)
pacificTime = utc.astimezone(to_zone)
today = datetime.now()
if today.date() == pacificTime.date():
parsedListOfCalendarEntryTitles.append("You have " + entry.title + " at " + pacificTime.strftime("%I:%M %p"))
print(parsedListOfCalendarEntryTitles)
python snips
python snips
edited Nov 16 '18 at 7:13
JeffC
asked Nov 16 '18 at 3:39
JeffCJeffC
62
62
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331076%2finsertion-sort-python-to-create-a-list-with-two-dependent-variables%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53331076%2finsertion-sort-python-to-create-a-list-with-two-dependent-variables%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
L9X e,3WpbUgCF4U3b,K4HPeQYeetQY826 MMJArd8ze QKdjnhJ,jCXhbuOr42T,OPGoqQ9Cj4SwNEG